Calling an external API in a form
When designing a form, it can happen you want to retrieve or verify data based on your customer input.
The easiest way is often to perform an API call to an external service then exploit its response to move forward with the rest of the form.
How to?
You will probably need to use two features in Penbox:
- The
api
element to specify the API call you want to perform & how to use its response - The
secrets store
available in your admin parameter via the Penbox plateform to drop the secret for your different external service in total security
API element documentation
The API element is currently not available via the Studio UI mode (coming soon)
{
"key": "data.my_api_call",
"type": "api",
"label": "Click me",
"url": "https://super-url",
"method": "GET",
"headers": {},
"body": {},
"mapping": {
"data.my_data_to_override": {
"value": "{@payload.my_data_to_override}",
"replace": true
},
"data.my_data_to_prefill": {
"value": "{@payload.my_data_to_prefill}",
"replace": false
}
}
}
- label : Label of the button to trigger the API call
- url: URL of the API service, variable(s) can of course be used to make the URL dynamic
- method: The standard HTTP methods: POST, PUT, PATCH, GET
- headers: The standard way of specifying HTTP headers (generally used for API authentication)
- body: The standard way of specifying HTTP body
- mapping: List of the data in your form you want to prefill / override
- replace: Determine if the data must be overriden if alreayd provided
- @payload is the variable containing the response data from the API call
Secret store documentation
-
Add a new secret to authenticate with a external service (as an API for instance)
-
Go to
Parameters/Secrets
-
Click the top right button
Add a secret
-
Specify a key so you can reference the secret you want to use later in your form
-
Specify your secret key or token (ATTENTION: it will never be visible anymore once created, you must remember it or store it safely elsewhere)
-
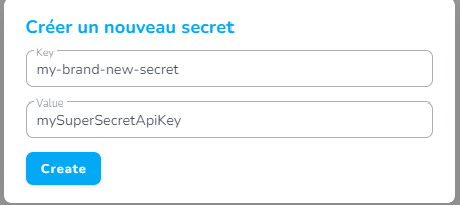
Real life example
As an insurer, I need to retrieve all legal information about my French corporate customer including:
- Official name of enterprise
- Legal form
- Number of employees
- ...
I want to propose a simplified user experience for my customer allowing him to only reference his SIRET code (FR enterprise number) and to prefill all other pieces of information for him to confirm in a click. To do so I will interrogate the API of a French enterprise legal information database : Pappers (cf. https://www.pappers.fr/)
How to do the magic?
- Create an account via the Pappers plateform and obtain a API token
- Create an new Penbox secret called :
pappers_api_key
- Create a form with all the questions you need to capture about the enterprise legal information
- Switch to
Script mode
to insert an API element before the questions create at step 3
Here is the working pen-script code 🤓
For the sake of the tuto, I set-up the authentication via the headers, but the Pappers API way of providing the secret is via the URL parameter "api_token"
[
{
"label": "Your enterprise information",
"elements": [
{
"key": "data.company_siret",
"max": 14,
"min": 14,
"mask": "##############",
"type": "text",
"title": {
"help": "By clicking the \"Check SIRET\" we will verify against our legal database all the legal information related to your enterprise.",
"text": "SIRET",
"inline": false
},
"required": true
},
{
"key": "data.siret_api_call",
"label": "Check SIRET",
"type": "api",
"method": "GET",
"url": "https://api.pappers.fr/v1/entreprise?siren={data.company_siret}",
"headers": {
"Authorization": "{$secrets.pappers_api_key}"
},
"mapping": {
"user.company_name": {
"value": "{@payload.nom_entreprise}",
"replace": true
},
"data.company_legal_form": {
"value": "{@payload.forme_juridique}",
"replace": true
},
"data.company_number_employee": {
"value": "{@payload.effectif_min}",
"replace": true
}
}
},
{
"key": "user.company_name",
"type": "text",
"title": "Company name"
},
{
"key": "data.company_legal_form",
"type": "text",
"title": "Company legal form"
},
{
"key": "data.company_number_employee",
"type": "text",
"title": "Company number of employees"
}
]
}
]
Enjoy the travel 🚀
Updated 4 months ago